Email Validation in PHP: A Comprehensive Guide
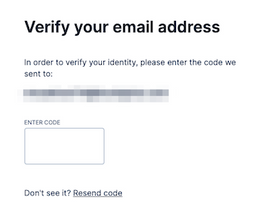
In the world of web development, ensuring that user input is accurate and secure is paramount. Among various types of user input, email addresses stand out due to their importance in communication and account management. This article delves into the intricacies of email validation in PHP, exploring various methods, best practices, and examples. By the end, you’ll have a solid understanding of how to implement effective email validation in your PHP applications.
Why Email Validation Matters
Email validation is the process of verifying that an email address is correctly formatted and likely exists. This is crucial for several reasons:
- User Experience: Users expect to receive confirmation emails and other communications. Invalid email addresses lead to frustration and loss of trust.
- Data Integrity: Storing invalid email addresses clutters your database and can lead to issues when sending newsletters or notifications.
- Spam Prevention: Email validation can help reduce the risk of spam registrations, contributing to a cleaner user base.
The Basics of Email Validation
Before diving into PHP-specific techniques, it’s essential to understand the basic structure of an email address. A valid email address typically follows the format local-part@domain
.
- Local Part: The part before the “@” symbol, which can include letters, numbers, and certain special characters.
- Domain Part: The part after the “@” symbol, which usually consists of a domain name followed by a top-level domain (TLD), such as
.com
,.org
, or.net
.
PHP Functions for Email Validation
PHP offers several built-in functions and libraries for email validation. Here are some common methods:
1. Using filter_var()
The simplest and most reliable way to validate an email address in PHP is to use the filter_var()
function with the FILTER_VALIDATE_EMAIL
filter.
$email = "test@example.com";
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "$email is a valid email address.";
} else {
echo "$email is not a valid email address.";
}
Advantages:
- Simplicity: It’s straightforward to use and understand.
- Performance: It is built into PHP, making it efficient.
2. Regular Expressions
Regular expressions (regex) offer a flexible way to validate email addresses by checking the format against a specific pattern.
$email = "test@example.com";
$pattern = "/^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/";
if (preg_match($pattern, $email)) {
echo "$email is a valid email address.";
} else {
echo "$email is not a valid email address.";
}
Advantages:
- Customizability: You can create complex patterns to suit specific requirements.
- Flexibility: Allows for advanced validation scenarios.
Disadvantages:
- Complexity: Regular expressions can be hard to read and maintain.
- Overhead: More code can lead to performance overhead.
Best Practices for Email Validation in PHP
To ensure effective email validation, consider the following best practices:
- Client-Side Validation: Implement JavaScript validation to provide instant feedback to users. This enhances user experience and reduces server load.
- Server-Side Validation: Always validate email addresses on the server side, even if client-side validation is implemented. This ensures security and data integrity.
- Use Multiple Validation Methods: Combine methods such as
filter_var()
and regex for comprehensive validation. This minimizes false positives and ensures accuracy. - Send Verification Emails: After registration, send a verification email to confirm that the user has access to the email address. This step adds an additional layer of validation.
- Handle Errors Gracefully: Provide clear error messages to users when validation fails. This helps users understand what went wrong and how to correct it.
Implementing Email Validation in a PHP Application
Let’s walk through an example of implementing email validation in a simple PHP registration form.
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$email = $_POST['email'];
$errors = [];
// Validate email format
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
$errors[] = "$email is not a valid email address.";
}
// Check for existing email (this would typically involve a database query)
$existingEmails = ["test@example.com", "hello@world.com"];
if (in_array($email, $existingEmails)) {
$errors[] = "$email is already registered.";
}
// Output results
if (empty($errors)) {
echo "Registration successful! A verification email has been sent to $email.";
} else {
foreach ($errors as $error) {
echo $error . "<br>";
}
}
}
<form method="post">
Email: <input type="text" name="email">
<input type="submit" value="Register">
</form>
Understanding the Code
- Form Handling: The code checks if the request method is POST, indicating the form has been submitted.
- Email Validation: The email is validated using
filter_var()
. If it fails, an error is added to the$errors
array. - Duplicate Check: A hypothetical array of existing emails simulates a database check to prevent duplicate registrations.
- Output: If there are no errors, a success message is displayed; otherwise, error messages are shown.
Advanced Email Validation Techniques
For developers looking for more robust validation options, consider integrating third-party libraries and services.
- Email Verification APIs: Services like ZeroBounce, Hunter, or NeverBounce can provide real-time validation and check if the email address is deliverable.
- DNS Lookup: Implementing a DNS lookup can verify if the domain part of the email address exists.
$domain = substr(strrchr($email, "@"), 1);
if (checkdnsrr($domain, "MX")) {
echo "$domain has valid MX records.";
} else {
echo "$domain does not have valid MX records.";
}
Common Pitfalls in Email Validation
- Overly Strict Validation: While it’s important to validate emails, being too strict can prevent valid addresses from being accepted. For instance, some valid email addresses may contain unusual characters or longer TLDs.
- Neglecting User Experience: Avoid making the validation process frustrating for users. Instead, provide clear feedback and instructions on how to enter their email addresses correctly.
- Ignoring Security Risks: While validation reduces the risk of invalid entries, it’s crucial to implement additional security measures, such as rate limiting and CAPTCHA, to prevent abuse.
Conclusion
Email validation in PHP is a critical component of web development that enhances user experience, data integrity, and security. By utilizing built-in functions, regular expressions, and following best practices, developers can ensure accurate and efficient validation of email addresses. Furthermore, considering advanced techniques and being mindful of common pitfalls will contribute to building robust applications that users can trust.
Implementing effective email validation will not only help in maintaining a clean database but also foster trust and reliability with your users. Always remember to combine various methods and approaches to create a comprehensive solution that meets the needs of your web applications.